MD5 Hash Generator - MD5 Hasher
Easily create an MD5 hash from a string with our free online MD5 hash generator tool.
An MD5 hash generator is a tool that is used to generate a hash value from a given input, typically a string of characters. The MD5 hash function is a widely used cryptographic hash function that produces a 128-bit hash value. The resulting hash is a unique representation of the input data, and any change to the input will result in a different hash value.
Why MD5 Hash is used?
One of the primary purposes of an MD5 hash generator is to ensure the integrity of data. For example, if you have a file that you want to download from a website, you can use an MD5 hash generator to generate a hash of the file before and after the download. If the two hashes are the same, then the file was downloaded successfully and has not been tampered with.
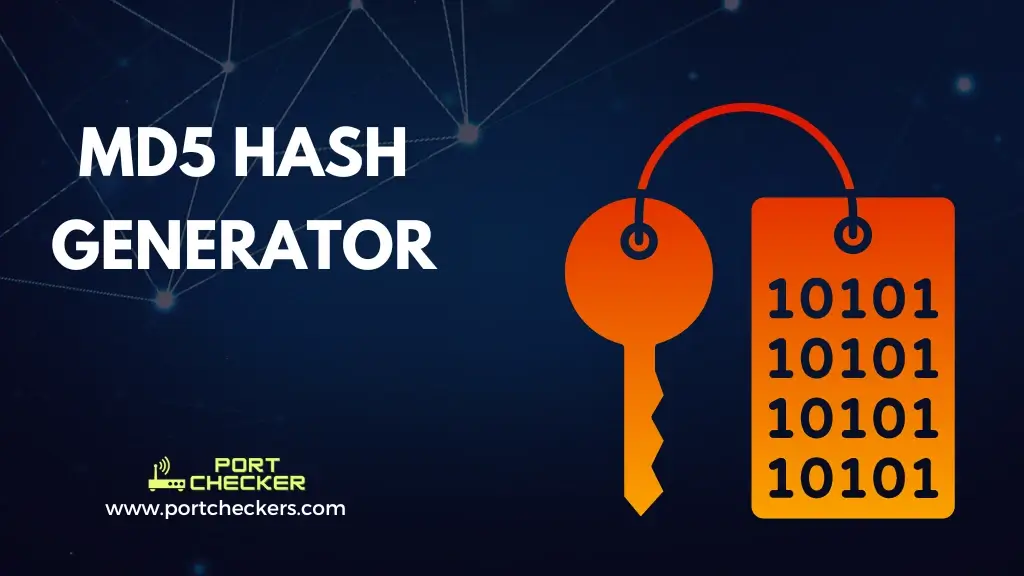
Another use of an MD5 hash generator is for storing passwords. When a user creates a password, the password can be hashed using an MD5 hash generator and the resulting hash value can be stored in a database instead of the actual password. This allows the password to be stored securely and protects it from being accessed by unauthorized users.
It is important to note that the MD5 algorithm is considered to be weak and should not be used for secure hashing. If you need a secure hash function, consider using a stronger algorithm such as SHA-256 or SHA-3.
Generating MD5 Hash Programmatically
-
Generate MD5 Hash in C#
using System.Security.Cryptography; public static string GenerateHash(string input) { using (MD5 md5Hash = MD5.Create()) { // ComputeHash - returns byte array byte[] bytes = md5Hash.ComputeHash(Encoding.UTF8.GetBytes(input)); // Convert byte array to a string StringBuilder builder = new StringBuilder(); for (int i = 0; i < bytes.Length; i++) { builder.Append(bytes[i].ToString("x2")); } return builder.ToString(); } }
-
Generate MD5 Hash in Python
import hashlib def generate_hash(input): return hashlib.md5(input.encode('utf-8')).hexdigest()
-
Generate MD5 Hash in PHP
function generate_hash($input) { return md5($input); }
-
Generate MD5 Hash in Java / Android
import java.math.BigInteger; import java.security.MessageDigest; public static String generateHash(String input) throws Exception { MessageDigest md = MessageDigest.getInstance("MD5"); byte[] messageDigest = md.digest(input.getBytes()); // Convert byte array into signum representation BigInteger no = new BigInteger(1, messageDigest); // Convert message digest into hex value String hashtext = no.toString(16); while (hashtext.length() < 32) { hashtext = "0" + hashtext; } return hashtext; }
-
Generate MD5 Hash in Javascript / Node Js
function generateHash(input) { const crypto = require('crypto'); return crypto.createHash('md5').update(input).digest('hex'); }